Python supports adding different arguments after adding its program name. These arguments are the command-line arguments, and Python has three ways you can handle these command-line arguments depending on what tasks you want to execute or how you want to handle the arguments. This post shares three main ways of working with the command-line arguments. Read on!
How to Work with Python Command Line Arguments
When running a Python script, you will likely supply parameters after the program’s name. These parameters are the command-line arguments, and they help you achieve different options within your programs.
Here are three ways of handling Python command-line arguments. We’ve discussed each and given examples to help you get comfortable with it.
Option 1: Working with sys Module
Python has the sys module, which takes the command-line arguments and stores them in a list. You can access the saved arguments using the sys.argv option and perform other tasks within your Python program.
There are three main features of using the sys module. You can store the command-line arguments into a list, get the length of the provided command-line arguments, or retrieve the name of the executed Python script.
In this example, we have a Python script that imports the Python sys module. It then prints out the name of the executed script, captures the provided arguments and stores them in a list. Lastly, it runs a for loop to display all the added command-line arguments to the output.
Once we execute the Python script, we get the following output. This output demonstrates the first way of working with Python command-line arguments.
Option 2: Using the getopt Module
The next approach to working with Python command-line arguments is using the getopt module. You will notice that its functionality is similar to the getopt function in the C programming language. With the getopt Python module, instead of storing the provided parameters into a list, it extends their separation. Thus, you can have a short form and long form version of the argument, and they will still be processed correctly.
For instance, typing the -h or –help as your command-line arguments will still be interpreted as the same parameters. When using the getopt module, you must also import the sys module to store the provided parameters.
Note: When working with the getopt module, remove the first stored element from your list using the sys.argv[1:] line.
In this example, we have imported the two modules, removed the first element in our list, set the short and long-form options to be matched, and parsed the arguments with the getopt module. Lastly, we have added the for loop to print different messages depending on the supplied parameters in its short or long form.
When we run the Python script, we can supply the short or long-form options, and the program will interpret them correctly.
Option 3: Using argparse Module
The last way of handling Python command-line arguments is using the argparse module. It is the better option among the three options as it has more ways options that you can use within your program. For instance, it helps you display help messages, and you can specify the data type of the argument.
Below is an example showing the basic way of working with the argparse module and it displays the -h option for showing the help message.
Once you run the Python program with the -h option, it will display a message like the one below.

You can also add a description to the help message displayed with the argsparse module by adding a new variable like in the code below.

Running the same Python program with the -h option, we now get a description for the help message.

You can take it further and define optional values for handling the command-line arguments. For instance, we have defined the -o option that prints a message if we supply it as a parameter.
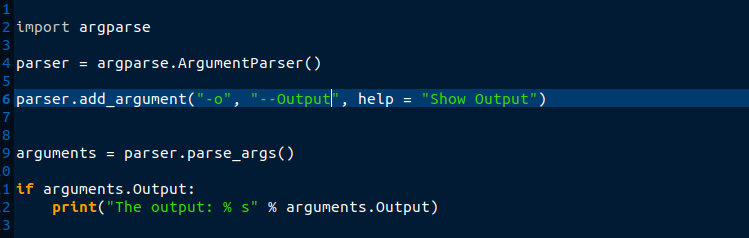
However, if you supply a different parameter not defined within our program, it throws an error as the argument is unrecognized.
Conclusion
Like other programming languages, Python allows users to supply command-line arguments as parameters for the executed script. There are three ways of handling Python command-line arguments, and this post has discussed each option in detail, giving practical examples. That’s it!